Simple Walkthrough on Visual Studio Code Snippets
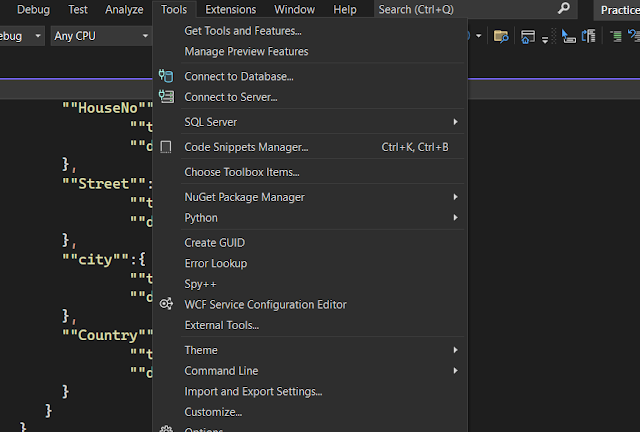
I guess most of the Software Developers might have used or aware of the Code Snippet feature in Visual Studio which helps us to write code much faster. Did any of us try to explore how Code Snippets work or in other words how the shortcut gets converted to extensive code? Let’s explore in this post how Code Snippet work and try to see where the code is defined for a particular Code Snippet. Exploring Existing Code Snippets First lets try to see all the existing snippets which comes by default in Visual Studio. This can be seen by opening the Code Snippets Manager by invoking the shortcut key(CTRL + K,CTRL + B) or go to Tools -> Code Snippets Manager as shown in the figure below: Once the Code Snippets Manager comes up, it will list down the available Code Snippets which are preloaded with the manager as shown below: Locating Code Snippet File To Understand the structure of the Code Snippet let us try...